🖥️ IT, 컴퓨터/🐍 Python
[Python] 구글 지도 위에 마커 올리기 (gmplot과 Google Maps JavaScript API 이용)
김 홍시
2025. 1. 17. 17:17
반응형
준비물
Google API
- Google Maps JavaScript API를 활성화해야 합니다.
- API 라이브러리에서 Maps JavaScript API를 검색하여 활성화하세요.
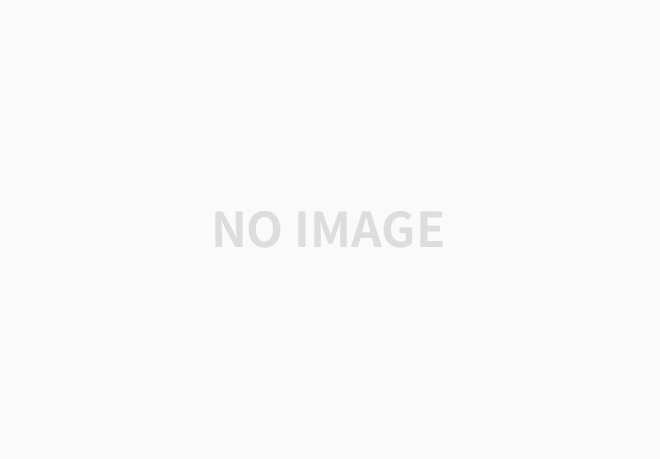
사용 클릭
import pandas as pd
from gmplot import gmplot
# CSV 파일 읽기
data = pd.read_csv('roi.csv')
# 위도와 경도 열 가져오기
latitudes = data['lat']
longitudes = data['lng']
# Google Maps 초기화 (중심점 설정)
gmap = gmplot.GoogleMapPlotter(latitudes.mean(), longitudes.mean(), 13, apikey='YOUR_API_KEY')
# 좌표에 마커 표시
gmap.scatter(latitudes, longitudes, color='red', size=40, marker=True)
# HTML 파일 생성
gmap.draw("map.html")
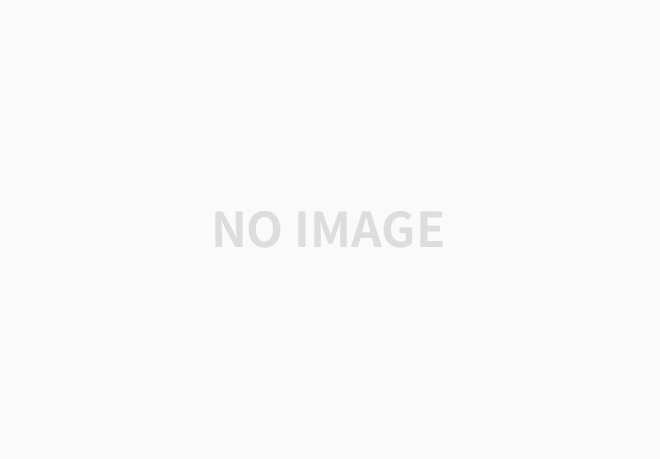
gmplot
을 사용하여 마커의 색상과 모양을 변경하는 방법은 아래와 같습니다. gmplot
에서 마커는 기본적으로 scatter
메서드로 그리지만, 개별 마커에 대해 색상과 모양을 변경하려면 HTML을 생성할 때 아이콘 URL을 사용하는 방법을 활용할 수 있습니다.
1. 색상 변경
gmplot.scatter
메서드에서 color
매개변수를 변경하면 마커의 색상을 변경할 수 있습니다.
코드 예시:
# 빨간색 마커를 파란색으로 변경
gmap.scatter(latitudes, longitudes, color='blue', size=40, marker=True)
2. 모양 변경
gmplot
에서 기본 제공되는 마커 모양은 동그라미뿐이므로, 특정 아이콘을 사용하려면 HTML 마커 아이콘을 직접 설정해야 합니다.
HTML 기반 커스텀 아이콘 추가 방법:
from gmplot import gmplot
import pandas as pd
# CSV 파일 읽기
data = pd.read_csv('roi.csv')
# Google Maps 초기화
gmap = gmplot.GoogleMapPlotter(data['lat'].mean(), data['lng'].mean(), 13, apikey='YOUR_API_KEY')
# 기본 마커 추가
gmap.scatter(data['lat'], data['lng'], color='blue', size=40, marker=True)
# HTML 파일 생성
gmap.draw("custom_map.html")
# HTML 파일 열기 및 수정 (커스텀 아이콘 추가)
with open("custom_map.html", "r", encoding="utf-8") as file:
html_content = file.read()
# 특정 위치의 아이콘 URL로 변경
custom_icon = """
var icon = {
url: "https://maps.google.com/mapfiles/kml/paddle/grn-circle.png",
scaledSize: new google.maps.Size(30, 30)
};
marker.setIcon(icon);
"""
# 마커에 커스텀 아이콘 설정 추가
html_content = html_content.replace("marker.setMap(map);", custom_icon + "\nmarker.setMap(map);")
# 업데이트된 HTML 저장
with open("custom_map.html", "w", encoding="utf-8") as file:
file.write(html_content)
주요 커스텀 아이콘 URL:
- Google Maps 기본 아이콘:
https://maps.google.com/mapfiles/kml/paddle/
- 예:
grn-circle.png
(녹색 원),red-diamond.png
(빨간 다이아몬드)
- 예:
- Custom 아이콘 추가:
다른 URL의 PNG 파일을 지정하여 원하는 모양의 아이콘을 사용할 수 있습니다.
--
반응형